GitHub offers numerous features. One of these is tracking of the deployments. When a new deployment is made, information about this is visible at first glance. It makes figuring out what is currently deployed easy and accessible.

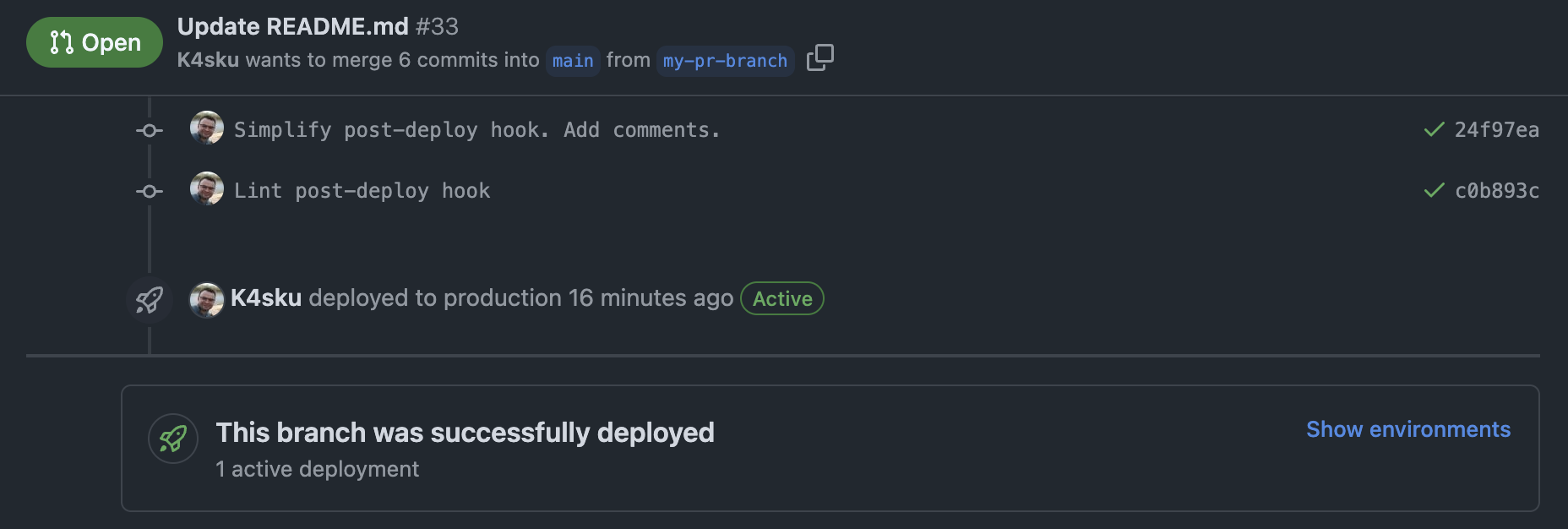
In order for GitHub to show this information, we have to notify it about a deployment. Every PaaS worth paying for has this feature. Since we are not paying Basecamp for using Kamal, we get none of this. So let's make it ourselves!
The plan
Use the Kamal Hooks mechanism to call GitHub's API using the official client: octokit
.
Creating a GitHub access token
Before implementation, we will need to create a GitHub token that will allow Kamal to use the API. Following the least privilege principle, we are using a fine-grained token scoped to a single repository with minimal required permissions.
Keep in mind that an organization can disallow using fine-grained personal access tokens or “classic” tokens. In this case you won’t be able to select repository.
To generate a token go to GitHub Settings > Developer Settings > Personal access tokens > Fine-grained tokens and click on “Generate new token”.
Give it a meaningful name. Select a repository and set repository permissions for Deployments
to Read and write
. The overview section on the bottom of the form should look like this:
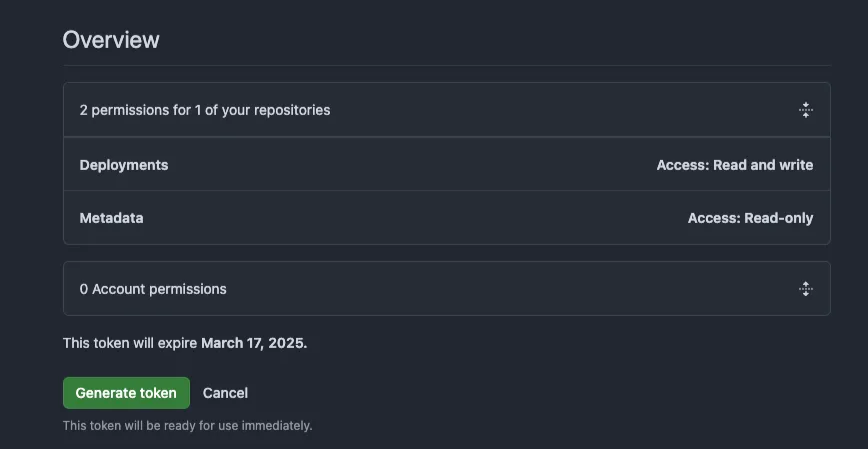
Note the token down, it will not be displayed again. I have added the token to .env file in the project as DEPLOYMENTS_GITHUB_TOKEN. If you do not use dotenv
you can set it in .bashrc
or however you config your environment.
Kamal hooks mechanism
During deployment process Kamal executes hooks. A hook is a script in a file matching a hook name. The file should have no extension and an execute file permission. When Kamal is added to the project, it creates sample hooks in <app root>/.kamal/hooks/
folder. At the time of writing this article there are 9 hooks available.
Hooks have access to deployment metadata via set of environment variables:
You can read more about hooks in the documentation.
Implementing the hook
With the plan, access and new knowledge we can start working on the implementation of the feature.
We are going to use a post-deploy
hook so we have to remove .sample
from the filename.
In the post-deploy hook we will:
- Install required
octokit
andfaraday-retry
gems. - Create a Deployment on GitHub API. New deployments always have state
queued
. The methods take two positional arguments: repository name and commit ref (branch, tag, or SHA). - Create a Deployment Status on GitHub API.It will update the Deployment with status
success
.
Final /.kamal/hooks/post-deploy
file:
Finale
With the hook set up we can finally deploy our application with:
Notice the dotenv
- it will load .env and pass DEPLOYMENTS_GITHUB_TOKEN
.
When the deploy ends you should see the deployment on GitHub repository.
With this simple ruby script deployments with Kamal are one step closer to the premium platform as a service experience.